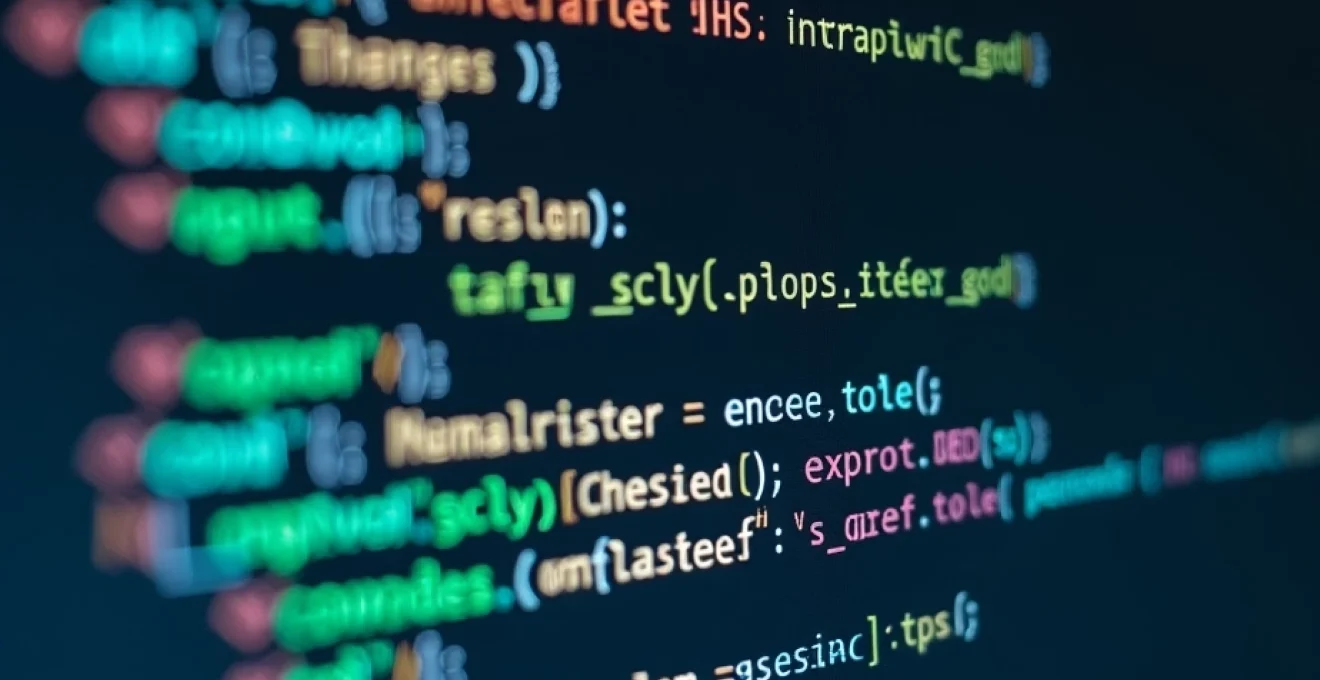
Web development is a dynamic and ever-evolving field that demands a diverse set of skills. As technology continues to advance, the role of web developers has become increasingly crucial in shaping the digital landscape. Whether you're just starting your journey or looking to enhance your existing skillset, mastering the essential web development skills is key to becoming a successful and sought-after professional in this competitive industry.
From front-end technologies that create stunning user interfaces to back-end systems that power complex applications, the world of web development offers endless opportunities for growth and innovation. In this comprehensive guide, we'll explore the core competencies and emerging trends that every aspiring web developer should focus on to stay ahead of the curve and build cutting-edge web solutions.
Mastering core programming languages for web development
At the heart of web development lies a solid foundation in core programming languages. These languages form the building blocks of every website and web application, enabling developers to create interactive and dynamic online experiences. To become a proficient web developer, it's essential to master the following key languages:
- HTML (Hypertext Markup Language)
- CSS (Cascading Style Sheets)
- JavaScript
- Python
- PHP
Each of these languages serves a specific purpose in web development. HTML provides the structure and content of web pages, CSS handles the visual styling and layout, while JavaScript adds interactivity and dynamic behavior. Python and PHP are popular server-side languages used for back-end development and data processing.
By gaining proficiency in these core languages, you'll have a solid foundation to build upon as you delve into more advanced web development concepts and technologies.
Front-end development essentials
Front-end development focuses on creating the user interface and user experience of websites and web applications. It's the part of web development that users directly interact with, making it crucial for delivering engaging and visually appealing online experiences. To excel in front-end development, you need to master several essential skills and technologies.
Advanced JavaScript techniques: closures, promises and Async/Await
JavaScript has evolved significantly over the years, introducing powerful features that enable developers to write more efficient and maintainable code. Understanding advanced JavaScript concepts is essential for creating robust front-end applications. Closures allow for data privacy and the creation of function factories. Promises provide a cleaner way to handle asynchronous operations, while the Async/Await syntax further simplifies asynchronous code, making it more readable and easier to reason about.
By mastering these advanced JavaScript techniques, you'll be able to write more elegant and performant code, handling complex scenarios with ease and improving the overall user experience of your web applications.
Mastering react.js: component lifecycle and hooks
React.js has become one of the most popular front-end libraries, revolutionizing the way developers build user interfaces. At the core of React's power lies its component-based architecture and efficient rendering mechanism. To truly master React, you need to understand the component lifecycle and the concept of hooks.
The component lifecycle refers to the different phases a React component goes through, from mounting to updating and unmounting. Understanding these phases allows you to optimize performance and manage side effects effectively. Hooks, introduced in React 16.8, provide a way to use state and other React features without writing class components, leading to more concise and reusable code.
By gaining expertise in React's component lifecycle and hooks, you'll be able to build more efficient and maintainable user interfaces, leveraging the full power of this popular library.
CSS3 and Sass: creating responsive layouts with Flexbox and Grid
Creating responsive and visually appealing layouts is a crucial skill for front-end developers. CSS3 introduced powerful layout systems like Flexbox and Grid, which have revolutionized the way we structure web pages. Flexbox provides a one-dimensional layout system for creating flexible and responsive designs, while Grid offers a two-dimensional system for more complex layouts.
Sass (Syntactically Awesome Style Sheets) is a CSS preprocessor that extends the capabilities of CSS, allowing for variables, nested rules, mixins, and functions. By combining CSS3 layout techniques with Sass, you can create more maintainable and scalable stylesheets, resulting in responsive designs that adapt seamlessly to different screen sizes and devices.
Typescript: enhancing JavaScript with static typing
TypeScript has gained significant popularity in recent years as a powerful superset of JavaScript that adds static typing and other advanced features. By using TypeScript, you can catch errors early in the development process, improve code quality, and enhance developer productivity.
Some key benefits of TypeScript include:
- Early detection of type-related errors
- Improved code maintainability and readability
- Better tooling and IDE support
- Enhanced refactoring capabilities
As more large-scale applications adopt TypeScript, becoming proficient in this language can significantly boost your value as a front-end developer and open up new career opportunities.
Back-end development fundamentals
While front-end development focuses on the user interface, back-end development deals with the server-side logic, databases, and APIs that power web applications. Mastering back-end development skills is crucial for building robust, scalable, and secure web solutions.
Node.js and Express.js: building RESTful APIs
Node.js has revolutionized back-end development by allowing JavaScript to run on the server-side. Its event-driven, non-blocking I/O model makes it ideal for building scalable and high-performance web applications. Express.js, a minimal and flexible Node.js web application framework, has become the de facto standard for building RESTful APIs.
Learning to create RESTful APIs with Node.js and Express.js is essential for modern web development. These APIs serve as the backbone for communication between front-end applications and back-end services, enabling the creation of dynamic and data-driven web experiences.
Database management: SQL vs. NoSQL (MongoDB, PostgreSQL)
Effective data management is crucial for any web application. As a back-end developer, you need to understand different database paradigms and choose the right solution for your project. The two main categories of databases are SQL (relational) and NoSQL (non-relational).
SQL databases like PostgreSQL offer strong consistency, complex querying capabilities, and are ideal for applications with structured data and complex relationships. NoSQL databases like MongoDB provide flexibility, scalability, and are well-suited for applications with large amounts of unstructured or semi-structured data.
Understanding the strengths and weaknesses of different database systems will allow you to make informed decisions when designing the data layer of your web applications.
Server-side rendering with Next.js
Server-side rendering (SSR) has gained popularity as a technique to improve the performance and SEO of web applications. Next.js, a React-based framework, provides an excellent solution for implementing SSR in your projects. By rendering pages on the server and sending fully-formed HTML to the client, Next.js offers several benefits:
- Improved initial page load times
- Better SEO, as search engines can easily crawl the content
- Enhanced performance on low-powered devices
- Improved accessibility for users with JavaScript disabled
Mastering Next.js and server-side rendering techniques will enable you to create fast, SEO-friendly, and highly performant web applications that provide an excellent user experience across various devices and network conditions.
GraphQL: optimizing data queries and mutations
GraphQL has emerged as a powerful alternative to traditional REST APIs, offering more flexibility and efficiency in data fetching. As a query language for APIs, GraphQL allows clients to request exactly the data they need, reducing over-fetching and under-fetching of data.
Key benefits of GraphQL include:
- Reduced network overhead by fetching only required data
- Strongly typed schema, providing better documentation and type safety
- Ability to aggregate data from multiple sources in a single request
- Easier versioning and deprecation of API fields
By mastering GraphQL, you'll be able to build more efficient and flexible APIs, improving the overall performance and maintainability of your web applications.
Devops and deployment strategies
In today's fast-paced development environment, understanding DevOps principles and deployment strategies is crucial for web developers. DevOps practices help bridge the gap between development and operations, enabling faster and more reliable software delivery.
Containerization with docker and orchestration with kubernetes
Containerization has revolutionized the way applications are packaged, deployed, and scaled. Docker, the leading containerization platform, allows developers to package applications with all their dependencies into standardized units called containers. These containers can run consistently across different environments, from development to production.
Kubernetes, an open-source container orchestration platform, takes containerization to the next level by automating the deployment, scaling, and management of containerized applications. By mastering Docker and Kubernetes, you'll be able to:
- Ensure consistency across development, testing, and production environments
- Simplify application deployment and scaling
- Improve resource utilization and reduce infrastructure costs
- Enhance application reliability and fault tolerance
Continuous Integration/Continuous deployment (CI/CD) pipelines
CI/CD pipelines are essential for modern software development, enabling teams to deliver high-quality code more frequently and reliably. Continuous Integration involves automatically building and testing code changes as they are committed to version control. Continuous Deployment takes this a step further by automatically deploying validated changes to production.
Learning to set up and manage CI/CD pipelines using tools like Jenkins, GitLab CI, or GitHub Actions will allow you to:
- Detect and fix integration issues early in the development process
- Automate repetitive tasks, reducing human error and freeing up developer time
- Ensure consistent and reliable deployments
- Facilitate rapid iteration and feedback cycles
Cloud platforms: AWS, Google Cloud and Azure
Cloud platforms have become an integral part of modern web development, offering scalable and flexible infrastructure for hosting and managing applications. The three major cloud providers - Amazon Web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure - offer a wide range of services for computing, storage, networking, and more.
Familiarity with these cloud platforms is essential for web developers, as it enables you to:
- Design and implement scalable and resilient architectures
- Leverage managed services to reduce operational overhead
- Implement cost-effective solutions for hosting and running web applications
- Utilize advanced features like serverless computing and machine learning services
Web security and performance optimization
As web applications become increasingly complex and handle sensitive user data, ensuring security and optimizing performance are critical aspects of web development. Mastering these areas will help you build trustworthy and high-performing web applications.
OWASP Top 10: mitigating common web vulnerabilities
The Open Web Application Security Project (OWASP) Top 10 is a regularly updated list of the most critical web application security risks. As a web developer, it's essential to understand these vulnerabilities and implement proper mitigation strategies. Some of the key areas to focus on include:
- Injection attacks (SQL injection, XSS)
- Broken authentication and session management
- Sensitive data exposure
- XML External Entities (XXE)
- Broken access control
By addressing these common vulnerabilities, you can significantly improve the security posture of your web applications and protect user data from potential breaches.
HTTPS implementation and SSL/TLS certificates
Implementing HTTPS and properly configuring SSL/TLS certificates is crucial for securing the communication between clients and servers. HTTPS encrypts data in transit, preventing eavesdropping and man-in-the-middle attacks. Additionally, many modern web features require HTTPS to function, making it essential for providing a full-featured web experience.
Understanding how to obtain, install, and manage SSL/TLS certificates, as well as configuring web servers for HTTPS, is a vital skill for web developers in today's security-conscious environment.
Web performance metrics: core web vitals and lighthouse
Web performance has a direct impact on user experience, conversion rates, and search engine rankings. Google's Core Web Vitals are a set of specific factors that Google considers important in a webpage's overall user experience. These include:
- Largest Contentful Paint (LCP): Measures loading performance
- First Input Delay (FID): Measures interactivity
- Cumulative Layout Shift (CLS): Measures visual stability
Lighthouse, an open-source tool for improving web page quality, provides audits for performance, accessibility, progressive web apps, and more. By understanding and optimizing for these metrics, you can create faster, more responsive, and user-friendly web experiences.
Caching strategies: Browser, CDN and server-side caching
Implementing effective caching strategies is crucial for improving web application performance and reducing server load. Different types of caching can be employed at various levels:
- Browser caching: Storing static assets locally in the user's browser
- CDN caching: Distributing content across global server networks for faster access
- Server-side caching: Storing frequently accessed data in memory for quick retrieval
Understanding when and how to implement these caching strategies can significantly improve the performance and scalability of your web applications.
Emerging technologies and future trends
Staying ahead in web development requires keeping an eye on emerging technologies and future trends. By familiarizing yourself with these cutting-edge concepts, you'll be better prepared to innovate and adapt to the evolving landscape of web development.
Progressive Web Apps (PWAs): service workers and web app manifests
Progressive Web Apps (PWAs) blend the best of web and mobile apps, offering a native app-like experience through web technologies. Key features of PWAs include offline functionality, push notifications, and the ability to add the app to the home screen. Service Workers, a key technology behind PWAs, enable offline caching and background syncing, while Web App Manifests provide metadata for controlling how the app appears when installed.
By mastering PWA development, you can create web applications that offer a more engaging and responsive user experience across various devices and network conditions.
Webassembly: high-performance web applications
WebAssembly (Wasm) is a low-level assembly-like language that runs at near-native speed in web browsers. It allows developers to write high-performance code in languages like C++ or Rust and run it alongside JavaScript in web applications. WebAssembly opens up new possibilities for web development, including:
- Running complex computations in the browser
- Porting existing desktop applications to the web
- Creating high-performance games and multimedia applications
- Improving the performance of CPU-intensive tasks in web apps
As WebAssembly continues to mature, it's poised to play a significant role in the future of web development, enabling more powerful and performant web applications.
Jamstack architecture: static site generators and headless CMS
Jamstack (JavaScript, APIs, and Markup) is an architectural approach that decouples the frontend from the backend, resulting
in faster loading times, improved security, and better scalability. The key components of Jamstack architecture include:
- Static Site Generators (SSGs): Tools like Gatsby, Next.js, and Hugo that generate static HTML files from source content and templates
- Headless CMS: Content management systems that provide a backend for managing content but leave the frontend presentation to developers
- APIs: Third-party services or custom backends that provide dynamic functionality
Jamstack architecture offers several benefits:
- Improved performance due to serving pre-rendered static files
- Enhanced security with a reduced attack surface
- Better scalability and lower hosting costs
- Improved developer experience with a clear separation of concerns
By mastering Jamstack architecture, static site generators, and headless CMS systems, you'll be well-equipped to build modern, high-performance websites that offer excellent user experiences and are easier to maintain and scale.